Steps for Creating a Chat-GPT Powered Flutter Application
Here’s an example of a working Flutter application built using ChatGPT and Flutter.
Task: Building a Tic-Tac-Toe Game Application with Flutter
Prompt: Create a tic-tac-toe game application in Flutter. Write and explain the code briefly.
Here’s the generated code:
import 'package:flutter/material.dart';
void main() {
runApp(TicTacToeApp());
}class TicTacToeApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Tic Tac Toe',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: TicTacToeScreen(),
);
}
}class TicTacToeScreen extends StatefulWidget {
@override
_TicTacToeScreenState createState() => _TicTacToeScreenState();
}class _TicTacToeScreenState extends State<TicTacToeScreen> {
List<List<String>> _board = [];
String _currentPlayer = "";
bool _gameOver = false; @override
void initState() {
super.initState();
_initializeBoard();
} void _initializeBoard() {
_board = List.generate(3, (_) => List.filled(3, ''));
_currentPlayer = 'X';
_gameOver = false;
} void _makeMove(int row, int col) {
if (_board[row][col] == '' && !_gameOver) {
setState(() {
_board[row][col] = _currentPlayer;
_checkGameStatus(row, col);
_togglePlayer();
});
}
} void _checkGameStatus(int row, int col) {
// Check rows
for (int i = 0; i < 3; i++) {
if (_board[i][0] == _board[i][1] &&
_board[i][1] == _board[i][2] &&
_board[i][0] != '') {
_endGame();
return;
}
} // Check columns
for (int i = 0; i < 3; i++) {
if (_board[0][i] == _board[1][i] &&
_board[1][i] == _board[2][i] &&
_board[0][i] != '') {
_endGame();
return;
}
} // Check diagonals
if (_board[0][0] == _board[1][1] &&
_board[1][1] == _board[2][2] &&
_board[0][0] != '') {
_endGame();
return;
} if (_board[0][2] == _board[1][1] &&
_board[1][1] == _board[2][0] &&
_board[0][2] != '') {
_endGame();
return;
} // Check for a draw
bool isBoardFull = true;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (_board[i][j] == '') {
isBoardFull = false;
break;
}
}
}
if (isBoardFull) {
_endGame();
}
} void _togglePlayer() {
_currentPlayer = (_currentPlayer == 'X') ? 'O' : 'X';
} void _endGame() {
_gameOver = true;
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text('Game Over'),
content: Text('The game has ended.'),
actions: [
TextButton(
onPressed: () {
setState(() {
_initializeBoard();
Navigator.of(context).pop();
});
},
child: Text('Restart'),
),
],
);
},
);
} @override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Tic Tac Toe'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Player: $_currentPlayer',
style: TextStyle(fontSize: 24.0),
),
SizedBox(height: 20.0),
GridView.builder(
shrinkWrap: true,
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 3,
),
itemCount: 9,
itemBuilder: (BuildContext context, int index) {
int row = index ~/ 3;
int col = index % 3;
return GestureDetector(
onTap: () => _makeMove(row, col),
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.black),
),
child: Center(
child: Text(
_board[row][col],
style: TextStyle(fontSize: 48.0),
),
),
),
);
},
),
],
),
),
);
}
}
This code provides a simple implementation of a tic-tac-toe game in Flutter. Players can make moves, and the code checks for a winner or a tie.
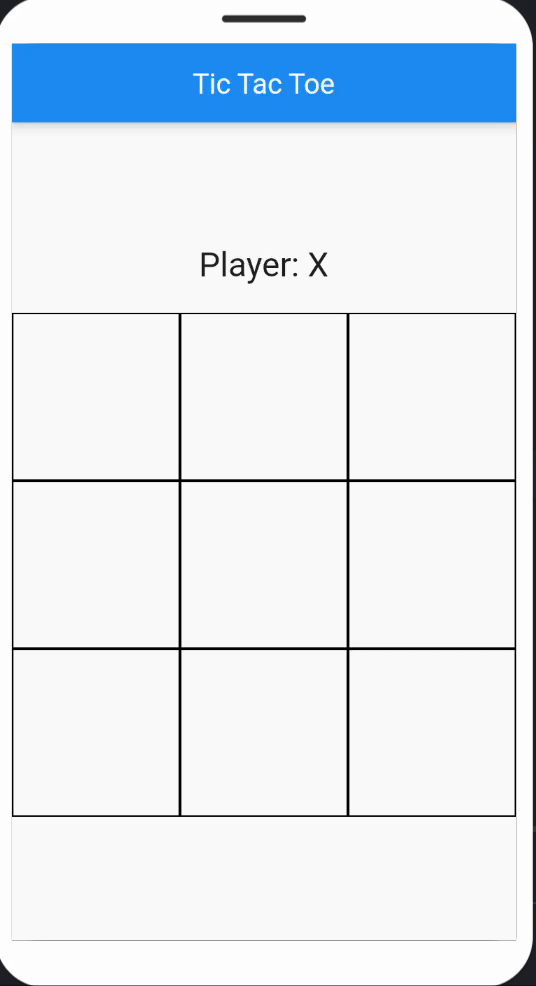
This is just one example of what you can do with ChatGPT and Flutter. To make this tic-tac-toe game more creative and engaging, we can integrate ChatGPT as the opponent!
Here is what you should ask chatGPT
Prompt: Create a creative tic-tac-toe application in Flutter. Where chatGPT is the opponent.
ChatGPT will explain how you can integrate it into your application using the application key.
void initializeChatGPT() {
// Initialize ChatGPT instance
chatGPT = OpenAIGPT3(
apiKey: 'YOUR_API_KEY',
defaultTemperature: 0.6,
defaultMaxTokens: 50,
);
}
In this case, you will get a worthy opponent (ChatGPT) who will have the ability to understand and respond to the player’s moves intelligently.
And voila, it’s that simple to create a Flutter application using ChatGPT!
Reference: https://blog.geekyants.com/how-flutter-and-chatgpt-will-change-app-development-86e7489d36d9
0 comments:
Post a Comment