Reference: https://c-nemri.medium.com/automate-your-reels-and-tiktoks-generation-using-python-faa4936159fe
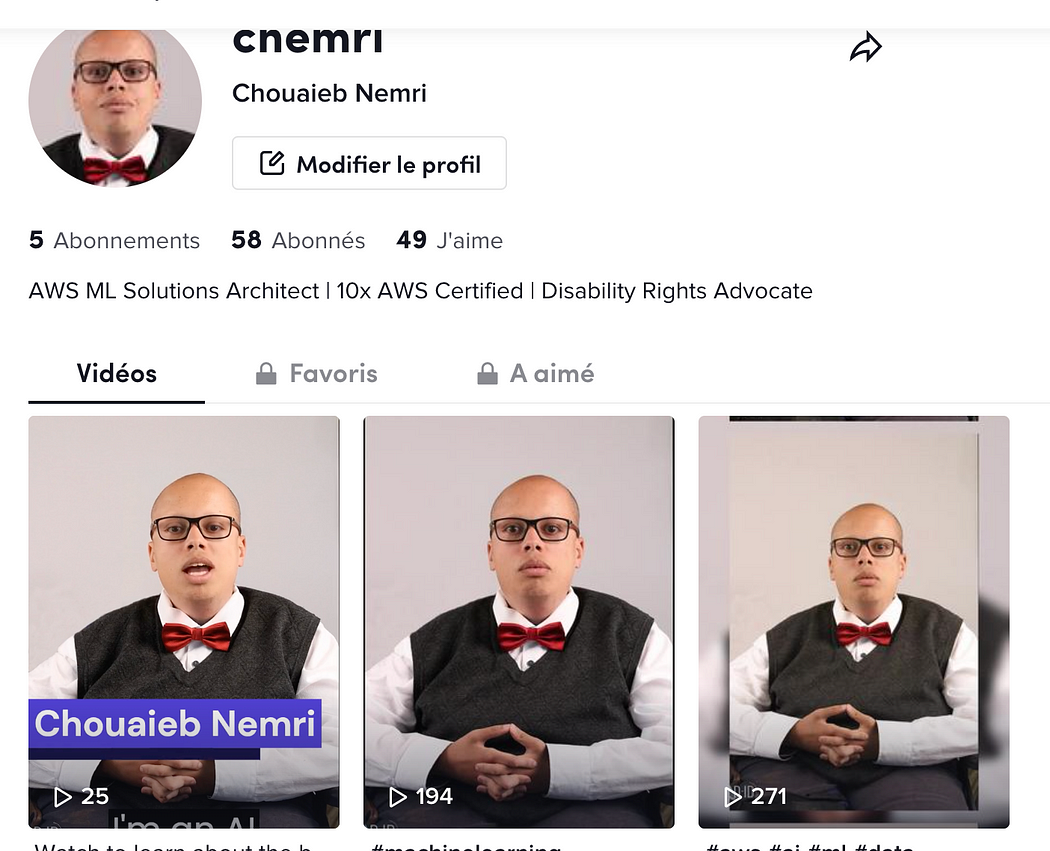
In this blog, I guide you through automating your video content generation using python and generative AI. By the end of this blog, you’ll be capable of:
- Create TikTok videos and Instagram reels featuring your virtual avatar
- Automatically generating a video script by just providing a 1-line idea to your content pipeline using OpenAI API.
- Automatically generating voice over for your video script using ElevenLabs API
- Automatically generate videos where your virtual avatar says your video script using D-ID API
Register to ElevenLabs and Create your Voice
- Go to ElevenLabs website and register (Starter plan is recommended if you want to clone your voice)
- Follow the following steps to clone your voice
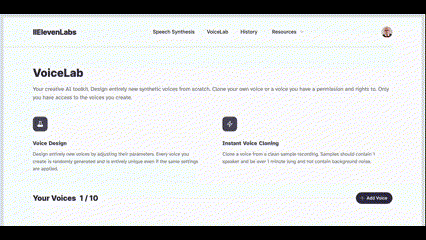
Register to D-ID and create your avatar
- Go to D-ID website and register (Lite plan is preferred to have a personal avatar)
- Follow the following steps to create your avatar
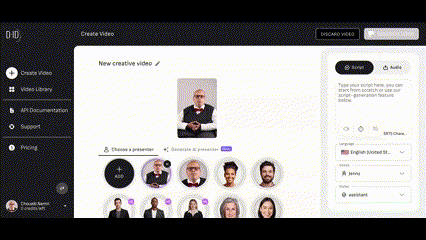
Register to OpenAI
- Register to OpenAI and create a new API key.
Automate your content pipeline using Python
Script generation
For script generation piece, we’ll use LangChain, which is a framework for developing applications powered by language models.
from langchain.llms import OpenAI
from langchain.prompts import PromptTemplate
from langchain.chains import LLMChain
llm = OpenAI(temperature=0)
template = """
You are the virtual avatar of Chouaieb Nemri. Given the following idea, write a 1 minute tiktok video script. Only write spoken words, not visuals. The speaker will be an AI generated avatar of myself.
Idea: {idea}
Script:
"""
prompt = PromptTemplate(
template=template,
input_variables=["idea"],
)
idea = """
Best Machine Learning course Andrew Ng's Machine Learning Specialization on Coursera
"""
chain = LLMChain(llm=llm, prompt=prompt)
script = chain.run(idea)
print(f"Generated script: {script}")
print("Is this script good? (y/n)")
user_input = input()
while user_input.lower() != "y":
script = chain.run(idea)
print(f"Generated script: {script}")
print("Is this script good? (y/n)")
user_input = input()
Audio Generation
We’ll use ElevenLabs python SDK to easily interact with their API. Code will look like the following.
from elevenlabs import generate, play, set_api_key, voices
voice_name = "Chouaieb"
print(f"Looking for voice id of {voice_name}")
voice_id = list(filter(lambda voice: voice.name == "Chouaieb", voices()))[0].voice_id
print(f"Found voice id: {voice_id}")
print(f"Generating audio with voice id: {voice_id}")
audio = generate(text=script, voice=voice_id, model="eleven_monolingual_v1")
with open("audio.mp3", "wb") as f:
f.write(audio)
Uploading Audio and avatar to Amazon S3
We’ll have to upload audio and avatar image to Amazon S3 and share the objects using Pre-signed URLs so that D-ID is able to access them and generate the video.
import boto3
bucket_name = "<YOUR BUCKET NAME>"
audio_file = "<YOUR AUDIO FILE NAME>"
image_file = "<YOUR IMAGE FILE NAME>"
session = boto3.Session(profile_name="personal")
s3_client = session.client('s3')
s3_client.upload_file(image_file, bucket_name, image_file)
s3_client.upload_file(audio_file, bucket_name, audio_file)
audio_url = s3_client.generate_presigned_url(
ClientMethod='get_object',
Params={
'Bucket': bucket_name,
'Key': audio_file
}
)
image_url = s3_client.generate_presigned_url(
ClientMethod='get_object',
Params={
'Bucket': bucket_name,
'Key': image_file
}
)
Generating and Downloading Video
We’ll use D-ID API to generate the video. The following code launches a video creation job, waits for it until it finishes, then downloads the video.
import requests
import datetime
url = "https://api.d-id.com/talks"
payload = {
"script": {
"type": "audio",
"reduce_noise": "false",
"audio_url": audio_url
},
"source_url": image_url,
"name": f"tiktok-video-{datetime.datetime.now().strftime('%Y-%m-%d-%H-%M-%S')}",
"config": {
"stitch": True
}
}
headers = {
"accept": "application/json",
"content-type": "application/json",
"authorization": "Basic <YOUR D-ID API KEY>"
}
print("Creating video...")
video_creation_response = requests.post(url, json=payload, headers=headers)
video_id = video_creation_response.json()['id']
url = f"https://api.d-id.com/talks/{video_id}"
video_get_response = requests.get(url, headers=headers)
while video_get_response.json()["status"] != "done":
video_get_response = video_get_response.get(url, headers=headers)
video_url = video_get_response.json()["result_url"]
r = requests.get(result_url, allow_redirects=True)
open("video.mp4", 'wb').write(r.content)
0 comments:
Post a Comment